Python program to convert a 24 hour format to 12 hour format
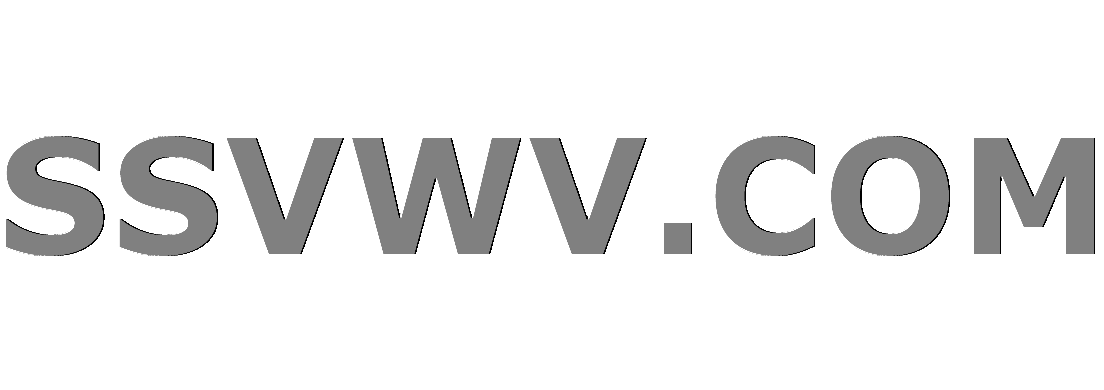
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
$begingroup$
Given a string in a 24 hour format the program outputs a 12 hour format. The rules are:
- The output format must be 'hh:mm a.m.' if it represents before midday and 'hh:mm p.m.' after midday
- When the hour is less than 10:00 you should not write a 0 before the hour, example: '9:05 a.m.'
For example:
12:30 → 12:30 p.m.
09:00 → 9:00 a.m.
23:15 → 11:15 p.m.
The code:
import datetime
def time_converter(time):
midday_dt = datetime.datetime.strptime('12:00','%H:%M')
time_dt = datetime.datetime.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= datetime.datetime.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < datetime.datetime.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= datetime.datetime.strptime('00:00','%H:%M') and
time_dt <= datetime.datetime.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
python beginner datetime formatting
$endgroup$
add a comment |
$begingroup$
Given a string in a 24 hour format the program outputs a 12 hour format. The rules are:
- The output format must be 'hh:mm a.m.' if it represents before midday and 'hh:mm p.m.' after midday
- When the hour is less than 10:00 you should not write a 0 before the hour, example: '9:05 a.m.'
For example:
12:30 → 12:30 p.m.
09:00 → 9:00 a.m.
23:15 → 11:15 p.m.
The code:
import datetime
def time_converter(time):
midday_dt = datetime.datetime.strptime('12:00','%H:%M')
time_dt = datetime.datetime.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= datetime.datetime.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < datetime.datetime.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= datetime.datetime.strptime('00:00','%H:%M') and
time_dt <= datetime.datetime.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
python beginner datetime formatting
$endgroup$
add a comment |
$begingroup$
Given a string in a 24 hour format the program outputs a 12 hour format. The rules are:
- The output format must be 'hh:mm a.m.' if it represents before midday and 'hh:mm p.m.' after midday
- When the hour is less than 10:00 you should not write a 0 before the hour, example: '9:05 a.m.'
For example:
12:30 → 12:30 p.m.
09:00 → 9:00 a.m.
23:15 → 11:15 p.m.
The code:
import datetime
def time_converter(time):
midday_dt = datetime.datetime.strptime('12:00','%H:%M')
time_dt = datetime.datetime.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= datetime.datetime.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < datetime.datetime.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= datetime.datetime.strptime('00:00','%H:%M') and
time_dt <= datetime.datetime.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
python beginner datetime formatting
$endgroup$
Given a string in a 24 hour format the program outputs a 12 hour format. The rules are:
- The output format must be 'hh:mm a.m.' if it represents before midday and 'hh:mm p.m.' after midday
- When the hour is less than 10:00 you should not write a 0 before the hour, example: '9:05 a.m.'
For example:
12:30 → 12:30 p.m.
09:00 → 9:00 a.m.
23:15 → 11:15 p.m.
The code:
import datetime
def time_converter(time):
midday_dt = datetime.datetime.strptime('12:00','%H:%M')
time_dt = datetime.datetime.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= datetime.datetime.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < datetime.datetime.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= datetime.datetime.strptime('00:00','%H:%M') and
time_dt <= datetime.datetime.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
python beginner datetime formatting
python beginner datetime formatting
edited May 26 at 12:55


200_success
135k21 gold badges173 silver badges443 bronze badges
135k21 gold badges173 silver badges443 bronze badges
asked May 26 at 8:12
enoyenoy
3771 silver badge9 bronze badges
3771 silver badge9 bronze badges
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
$begingroup$
To help with readability, I'd just import datetime.datetime
and then alias it, that way you aren't typing datetime.datetime
all over the place:
from datetime import datetime as dt
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= dt.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < dt.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= dt.strptime('00:00','%H:%M') and
time_dt <= dt.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
Next, if you are just unpacking the list
returned from clamp_to_twelve
, I would just return a tuple
:
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return hours, minutes
This saves you from having to construct the list, as well as the additional overhead of over-allocating memory to take into account the mutable list
.
As a last optimization, you could refactor your if
statements to not be nested. Because you are using if if
rather than if elif
, all of your statements are executed within the nested blocks:
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= dt.strptime('13:00', '%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} p.m.'
elif time_dt > midday_dt:
time += ' p.m.'
elif time_dt < dt.strptime('10:00', '%H:%M'):
time = f'{time[1:]} a.m.'
elif is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} a.m.'
else:
time += ' a.m.'
return time
The only issue being that the order is quite important, since time_dt > midday_dt
and time_dt >= dt.strptime('13:00', '%H:%M')
are not mutually exclusive. However, you do get a slight time bump because of the separation of blocks of code:
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter(t) for t in times)'
1000000 loops, best of 3: 0.275 usec per loop
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter_2(t) for t in times)'
1000000 loops, best of 3: 0.277 usec per loop
Emphasis on slight. Depends on what you find more readable.
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "196"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f221042%2fpython-program-to-convert-a-24-hour-format-to-12-hour-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
To help with readability, I'd just import datetime.datetime
and then alias it, that way you aren't typing datetime.datetime
all over the place:
from datetime import datetime as dt
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= dt.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < dt.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= dt.strptime('00:00','%H:%M') and
time_dt <= dt.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
Next, if you are just unpacking the list
returned from clamp_to_twelve
, I would just return a tuple
:
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return hours, minutes
This saves you from having to construct the list, as well as the additional overhead of over-allocating memory to take into account the mutable list
.
As a last optimization, you could refactor your if
statements to not be nested. Because you are using if if
rather than if elif
, all of your statements are executed within the nested blocks:
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= dt.strptime('13:00', '%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} p.m.'
elif time_dt > midday_dt:
time += ' p.m.'
elif time_dt < dt.strptime('10:00', '%H:%M'):
time = f'{time[1:]} a.m.'
elif is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} a.m.'
else:
time += ' a.m.'
return time
The only issue being that the order is quite important, since time_dt > midday_dt
and time_dt >= dt.strptime('13:00', '%H:%M')
are not mutually exclusive. However, you do get a slight time bump because of the separation of blocks of code:
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter(t) for t in times)'
1000000 loops, best of 3: 0.275 usec per loop
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter_2(t) for t in times)'
1000000 loops, best of 3: 0.277 usec per loop
Emphasis on slight. Depends on what you find more readable.
$endgroup$
add a comment |
$begingroup$
To help with readability, I'd just import datetime.datetime
and then alias it, that way you aren't typing datetime.datetime
all over the place:
from datetime import datetime as dt
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= dt.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < dt.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= dt.strptime('00:00','%H:%M') and
time_dt <= dt.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
Next, if you are just unpacking the list
returned from clamp_to_twelve
, I would just return a tuple
:
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return hours, minutes
This saves you from having to construct the list, as well as the additional overhead of over-allocating memory to take into account the mutable list
.
As a last optimization, you could refactor your if
statements to not be nested. Because you are using if if
rather than if elif
, all of your statements are executed within the nested blocks:
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= dt.strptime('13:00', '%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} p.m.'
elif time_dt > midday_dt:
time += ' p.m.'
elif time_dt < dt.strptime('10:00', '%H:%M'):
time = f'{time[1:]} a.m.'
elif is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} a.m.'
else:
time += ' a.m.'
return time
The only issue being that the order is quite important, since time_dt > midday_dt
and time_dt >= dt.strptime('13:00', '%H:%M')
are not mutually exclusive. However, you do get a slight time bump because of the separation of blocks of code:
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter(t) for t in times)'
1000000 loops, best of 3: 0.275 usec per loop
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter_2(t) for t in times)'
1000000 loops, best of 3: 0.277 usec per loop
Emphasis on slight. Depends on what you find more readable.
$endgroup$
add a comment |
$begingroup$
To help with readability, I'd just import datetime.datetime
and then alias it, that way you aren't typing datetime.datetime
all over the place:
from datetime import datetime as dt
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= dt.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < dt.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= dt.strptime('00:00','%H:%M') and
time_dt <= dt.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
Next, if you are just unpacking the list
returned from clamp_to_twelve
, I would just return a tuple
:
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return hours, minutes
This saves you from having to construct the list, as well as the additional overhead of over-allocating memory to take into account the mutable list
.
As a last optimization, you could refactor your if
statements to not be nested. Because you are using if if
rather than if elif
, all of your statements are executed within the nested blocks:
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= dt.strptime('13:00', '%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} p.m.'
elif time_dt > midday_dt:
time += ' p.m.'
elif time_dt < dt.strptime('10:00', '%H:%M'):
time = f'{time[1:]} a.m.'
elif is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} a.m.'
else:
time += ' a.m.'
return time
The only issue being that the order is quite important, since time_dt > midday_dt
and time_dt >= dt.strptime('13:00', '%H:%M')
are not mutually exclusive. However, you do get a slight time bump because of the separation of blocks of code:
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter(t) for t in times)'
1000000 loops, best of 3: 0.275 usec per loop
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter_2(t) for t in times)'
1000000 loops, best of 3: 0.277 usec per loop
Emphasis on slight. Depends on what you find more readable.
$endgroup$
To help with readability, I'd just import datetime.datetime
and then alias it, that way you aren't typing datetime.datetime
all over the place:
from datetime import datetime as dt
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= midday_dt:
if time_dt >= dt.strptime('13:00','%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes}'
time += ' p.m.'
else:
if time_dt < dt.strptime('10:00','%H:%M'):
time = time[1:]
if is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes:02d}'
time += ' a.m.'
return time
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return [hours, minutes]
def is_midnight(time_dt):
return (time_dt >= dt.strptime('00:00','%H:%M') and
time_dt <= dt.strptime('00:59','%H:%M'))
if __name__ == '__main__':
assert time_converter('12:30') == '12:30 p.m.'
assert time_converter('09:00') == '9:00 a.m.'
assert time_converter('23:15') == '11:15 p.m.'
Next, if you are just unpacking the list
returned from clamp_to_twelve
, I would just return a tuple
:
def clamp_to_twelve(time_dt, midday_dt):
clamp_dt = time_dt - midday_dt
minutes, seconds = divmod(clamp_dt.seconds, 60)
hours, minutes = divmod(minutes, 60)
return hours, minutes
This saves you from having to construct the list, as well as the additional overhead of over-allocating memory to take into account the mutable list
.
As a last optimization, you could refactor your if
statements to not be nested. Because you are using if if
rather than if elif
, all of your statements are executed within the nested blocks:
def time_converter(time):
midday_dt = dt.strptime('12:00','%H:%M')
time_dt = dt.strptime(time, '%H:%M')
if time_dt >= dt.strptime('13:00', '%H:%M'):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} p.m.'
elif time_dt > midday_dt:
time += ' p.m.'
elif time_dt < dt.strptime('10:00', '%H:%M'):
time = f'{time[1:]} a.m.'
elif is_midnight(time_dt):
hours, minutes = clamp_to_twelve(time_dt, midday_dt)
time = f'{hours}:{minutes} a.m.'
else:
time += ' a.m.'
return time
The only issue being that the order is quite important, since time_dt > midday_dt
and time_dt >= dt.strptime('13:00', '%H:%M')
are not mutually exclusive. However, you do get a slight time bump because of the separation of blocks of code:
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter(t) for t in times)'
1000000 loops, best of 3: 0.275 usec per loop
python -m timeit -s "from file import time_converter, time_converter_2; times = ['12:30', '09:00', '11:15']" '(time_converter_2(t) for t in times)'
1000000 loops, best of 3: 0.277 usec per loop
Emphasis on slight. Depends on what you find more readable.
edited Jul 3 at 9:31


Toby Speight
31.1k7 gold badges45 silver badges135 bronze badges
31.1k7 gold badges45 silver badges135 bronze badges
answered Jul 2 at 20:59
C.NivsC.Nivs
3361 silver badge8 bronze badges
3361 silver badge8 bronze badges
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f221042%2fpython-program-to-convert-a-24-hour-format-to-12-hour-format%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uPmfExg 2,u6qQooZlYhwRPW3xRB VS,46ycrOHXpoJ,xlP6SE7t,5qr