C++ forcing function parameter evalution order
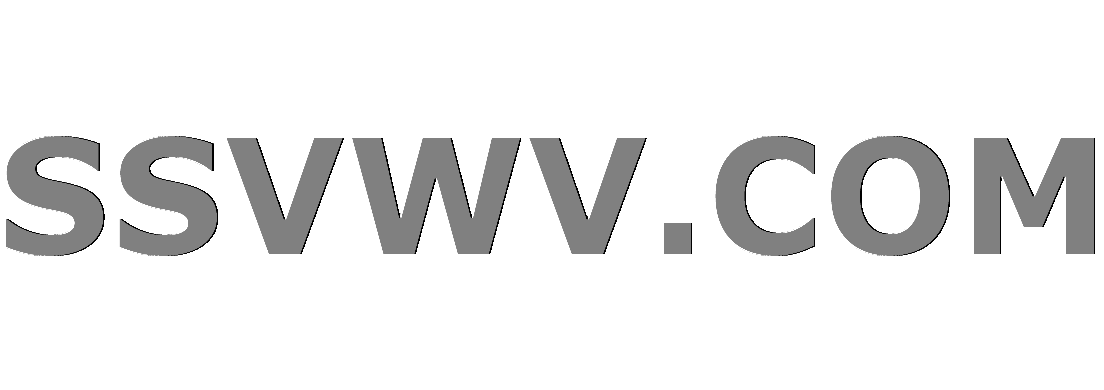
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ margin-bottom:0;
}
I understand that when I call a function such as
a(b(),c());
then the behavior of this may be undefined in <= C++14, and unspecified in >=C++17 in the sense that it is up to the compiler to determine whether to evaluate b
or c
first.
I would like to know the best way to force an evaluation order. I will be compiling as C++14.
The thing that immediately comes to mind is something like this:
#include <iostream>
int count = 5;
auto increment(){
return count++;
}
template <typename A, typename B>
auto diff(A && a, B && b){
return a - b;
}
int main() {
auto && a = increment();
auto && b = increment();
auto c = diff(a,b);
}
Am I in undefined behavior land? Or is this how one is "supposed" to force evaluation order?
c++ c++14
|
show 5 more comments
I understand that when I call a function such as
a(b(),c());
then the behavior of this may be undefined in <= C++14, and unspecified in >=C++17 in the sense that it is up to the compiler to determine whether to evaluate b
or c
first.
I would like to know the best way to force an evaluation order. I will be compiling as C++14.
The thing that immediately comes to mind is something like this:
#include <iostream>
int count = 5;
auto increment(){
return count++;
}
template <typename A, typename B>
auto diff(A && a, B && b){
return a - b;
}
int main() {
auto && a = increment();
auto && b = increment();
auto c = diff(a,b);
}
Am I in undefined behavior land? Or is this how one is "supposed" to force evaluation order?
c++ c++14
4
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
2
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
2
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
1
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
7
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified meansb()
and thenc()
, orc()
and thenb()
. One of them will happen. It is not specified, which.
– geza
May 24 at 14:14
|
show 5 more comments
I understand that when I call a function such as
a(b(),c());
then the behavior of this may be undefined in <= C++14, and unspecified in >=C++17 in the sense that it is up to the compiler to determine whether to evaluate b
or c
first.
I would like to know the best way to force an evaluation order. I will be compiling as C++14.
The thing that immediately comes to mind is something like this:
#include <iostream>
int count = 5;
auto increment(){
return count++;
}
template <typename A, typename B>
auto diff(A && a, B && b){
return a - b;
}
int main() {
auto && a = increment();
auto && b = increment();
auto c = diff(a,b);
}
Am I in undefined behavior land? Or is this how one is "supposed" to force evaluation order?
c++ c++14
I understand that when I call a function such as
a(b(),c());
then the behavior of this may be undefined in <= C++14, and unspecified in >=C++17 in the sense that it is up to the compiler to determine whether to evaluate b
or c
first.
I would like to know the best way to force an evaluation order. I will be compiling as C++14.
The thing that immediately comes to mind is something like this:
#include <iostream>
int count = 5;
auto increment(){
return count++;
}
template <typename A, typename B>
auto diff(A && a, B && b){
return a - b;
}
int main() {
auto && a = increment();
auto && b = increment();
auto c = diff(a,b);
}
Am I in undefined behavior land? Or is this how one is "supposed" to force evaluation order?
c++ c++14
c++ c++14
edited May 24 at 12:06
StoryTeller
116k18 gold badges250 silver badges316 bronze badges
116k18 gold badges250 silver badges316 bronze badges
asked May 24 at 9:40
bremen_mattbremen_matt
3,0932 gold badges22 silver badges45 bronze badges
3,0932 gold badges22 silver badges45 bronze badges
4
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
2
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
2
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
1
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
7
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified meansb()
and thenc()
, orc()
and thenb()
. One of them will happen. It is not specified, which.
– geza
May 24 at 14:14
|
show 5 more comments
4
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
2
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
2
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
1
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
7
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified meansb()
and thenc()
, orc()
and thenb()
. One of them will happen. It is not specified, which.
– geza
May 24 at 14:14
4
4
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
2
2
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
2
2
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
1
1
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
7
7
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified means
b()
and then c()
, or c()
and then b()
. One of them will happen. It is not specified, which.– geza
May 24 at 14:14
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified means
b()
and then c()
, or c()
and then b()
. One of them will happen. It is not specified, which.– geza
May 24 at 14:14
|
show 5 more comments
2 Answers
2
active
oldest
votes
The semi-colon that separates statements imposes a "happens before" relation.
auto && a = increment()
must be evaluated first. It is guaranteed. The returned temporary will be bound to the reference a
(and its lifetime extended) before the second call to increment
.
There is no UB. This is the way to force an evaluation order.
The only gotcha here is if increment
returned a reference itself, then you'd need to worry about lifetime issues. But if there was no lifetime issues, say if it returned a reference to count
, there still would not be UB from the imposed evaluation of a
and then b
.
add a comment |
Here's another way to force the evaluation order, using a std::initializer_list
, which has a guaranteed left-to-right order of evaluation:
#include <numeric> // for accumulate
#include <initializer_list>
template <class T>
auto diff(std::initializer_list<T> args)
{
return std::accumulate(args.begin(), args.end(), T(0), std::minus<>{});
}
const auto result = diff({increment(), increment()});
This restricts you to objects of the same type, and you need to type additional braces.
Not sure but I think you can get this to work with different types via tuples:std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:std::accumulate
doesstd::plus<>
by default, so we need to pass astd::minus<>
instance to do the actual subtraction.
– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56289880%2fc-forcing-function-parameter-evalution-order%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The semi-colon that separates statements imposes a "happens before" relation.
auto && a = increment()
must be evaluated first. It is guaranteed. The returned temporary will be bound to the reference a
(and its lifetime extended) before the second call to increment
.
There is no UB. This is the way to force an evaluation order.
The only gotcha here is if increment
returned a reference itself, then you'd need to worry about lifetime issues. But if there was no lifetime issues, say if it returned a reference to count
, there still would not be UB from the imposed evaluation of a
and then b
.
add a comment |
The semi-colon that separates statements imposes a "happens before" relation.
auto && a = increment()
must be evaluated first. It is guaranteed. The returned temporary will be bound to the reference a
(and its lifetime extended) before the second call to increment
.
There is no UB. This is the way to force an evaluation order.
The only gotcha here is if increment
returned a reference itself, then you'd need to worry about lifetime issues. But if there was no lifetime issues, say if it returned a reference to count
, there still would not be UB from the imposed evaluation of a
and then b
.
add a comment |
The semi-colon that separates statements imposes a "happens before" relation.
auto && a = increment()
must be evaluated first. It is guaranteed. The returned temporary will be bound to the reference a
(and its lifetime extended) before the second call to increment
.
There is no UB. This is the way to force an evaluation order.
The only gotcha here is if increment
returned a reference itself, then you'd need to worry about lifetime issues. But if there was no lifetime issues, say if it returned a reference to count
, there still would not be UB from the imposed evaluation of a
and then b
.
The semi-colon that separates statements imposes a "happens before" relation.
auto && a = increment()
must be evaluated first. It is guaranteed. The returned temporary will be bound to the reference a
(and its lifetime extended) before the second call to increment
.
There is no UB. This is the way to force an evaluation order.
The only gotcha here is if increment
returned a reference itself, then you'd need to worry about lifetime issues. But if there was no lifetime issues, say if it returned a reference to count
, there still would not be UB from the imposed evaluation of a
and then b
.
edited May 24 at 21:09
Fabio Turati
2,8565 gold badges25 silver badges43 bronze badges
2,8565 gold badges25 silver badges43 bronze badges
answered May 24 at 9:43
StoryTellerStoryTeller
116k18 gold badges250 silver badges316 bronze badges
116k18 gold badges250 silver badges316 bronze badges
add a comment |
add a comment |
Here's another way to force the evaluation order, using a std::initializer_list
, which has a guaranteed left-to-right order of evaluation:
#include <numeric> // for accumulate
#include <initializer_list>
template <class T>
auto diff(std::initializer_list<T> args)
{
return std::accumulate(args.begin(), args.end(), T(0), std::minus<>{});
}
const auto result = diff({increment(), increment()});
This restricts you to objects of the same type, and you need to type additional braces.
Not sure but I think you can get this to work with different types via tuples:std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:std::accumulate
doesstd::plus<>
by default, so we need to pass astd::minus<>
instance to do the actual subtraction.
– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
add a comment |
Here's another way to force the evaluation order, using a std::initializer_list
, which has a guaranteed left-to-right order of evaluation:
#include <numeric> // for accumulate
#include <initializer_list>
template <class T>
auto diff(std::initializer_list<T> args)
{
return std::accumulate(args.begin(), args.end(), T(0), std::minus<>{});
}
const auto result = diff({increment(), increment()});
This restricts you to objects of the same type, and you need to type additional braces.
Not sure but I think you can get this to work with different types via tuples:std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:std::accumulate
doesstd::plus<>
by default, so we need to pass astd::minus<>
instance to do the actual subtraction.
– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
add a comment |
Here's another way to force the evaluation order, using a std::initializer_list
, which has a guaranteed left-to-right order of evaluation:
#include <numeric> // for accumulate
#include <initializer_list>
template <class T>
auto diff(std::initializer_list<T> args)
{
return std::accumulate(args.begin(), args.end(), T(0), std::minus<>{});
}
const auto result = diff({increment(), increment()});
This restricts you to objects of the same type, and you need to type additional braces.
Here's another way to force the evaluation order, using a std::initializer_list
, which has a guaranteed left-to-right order of evaluation:
#include <numeric> // for accumulate
#include <initializer_list>
template <class T>
auto diff(std::initializer_list<T> args)
{
return std::accumulate(args.begin(), args.end(), T(0), std::minus<>{});
}
const auto result = diff({increment(), increment()});
This restricts you to objects of the same type, and you need to type additional braces.
edited May 27 at 12:35
answered May 24 at 9:45


lubgrlubgr
24.4k3 gold badges32 silver badges77 bronze badges
24.4k3 gold badges32 silver badges77 bronze badges
Not sure but I think you can get this to work with different types via tuples:std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:std::accumulate
doesstd::plus<>
by default, so we need to pass astd::minus<>
instance to do the actual subtraction.
– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
add a comment |
Not sure but I think you can get this to work with different types via tuples:std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:std::accumulate
doesstd::plus<>
by default, so we need to pass astd::minus<>
instance to do the actual subtraction.
– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
Not sure but I think you can get this to work with different types via tuples:
std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
Not sure but I think you can get this to work with different types via tuples:
std::apply(func, std::tuple<funcsargs>({__VA_ARGS__}));
– sudo rm -rf slash
May 25 at 7:02
apply is C++17 I think
– bremen_matt
May 27 at 11:29
apply is C++17 I think
– bremen_matt
May 27 at 11:29
1
1
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:
std::accumulate
does std::plus<>
by default, so we need to pass a std::minus<>
instance to do the actual subtraction.– lubgr
May 27 at 12:35
@bremen_matt Thanks for the edit for consistency with the question. Minor nitpick:
std::accumulate
does std::plus<>
by default, so we need to pass a std::minus<>
instance to do the actual subtraction.– lubgr
May 27 at 12:35
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
Oops. Slipped through the cracks. I changed the example slightly so that it is easier for others to understand why order of execution is important. In the previous example, it actually didn't matter.
– bremen_matt
May 27 at 13:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f56289880%2fc-forcing-function-parameter-evalution-order%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7hXijY2knggjwYIZq vkgG zXTVQM,wfkl0hvX5q
4
why do you think there could be ub?
– formerlyknownas_463035818
May 24 at 9:42
2
@Peter even if a global variable is used (read and/or written) by the two functions, the behavior would not be undefined, but just unspecified.
– j6t
May 24 at 10:59
2
@Peter Expressions can be evaluated in an interleaved manner up to C++14, but even then each function invocation in an argument must be executed as a whole. It has never been the case that functions could have been executed interleaved or in parallel. See rule 11 in the Rules section (and rule 4 in the sequence point rules further down).
– j6t
May 24 at 11:23
1
@bremen_matt: diff doesn't make a difference (pun intended), it is still just unspecified, not UB. There is no difference between standard versions in this regard, this was always unspecified.
– geza
May 24 at 12:16
7
@bremen_matt: Undefined means anything can happen (the standard doesn't specify what should happen). Unspecified means
b()
and thenc()
, orc()
and thenb()
. One of them will happen. It is not specified, which.– geza
May 24 at 14:14