How can a C program poll for user input while simultaneously performing other actions in a Linux environment?
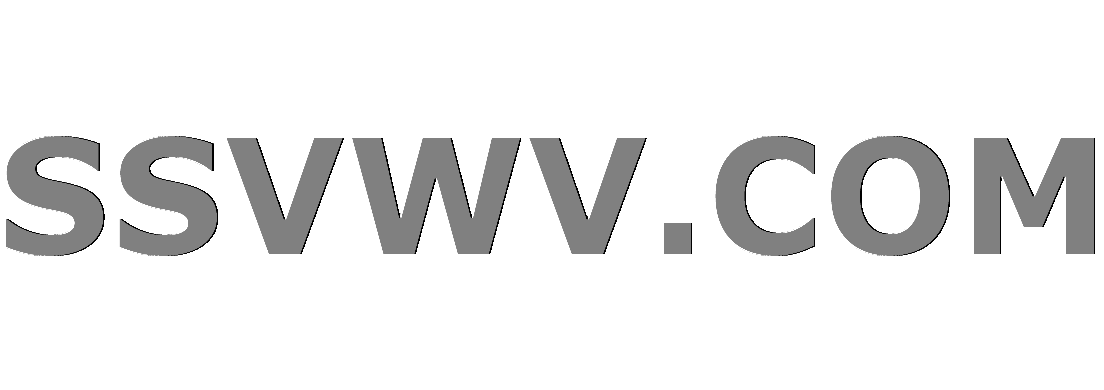
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Background:
I'm a relatively inexperienced developer trying to write software to interface with a PCI motion controller. I'm using C (compiled with gcc) on Ubuntu Linux 18.04.
The program I'm writing needs to regularly check for unsolicited status messages sent by the motion controller (approx. once per second) and display any messages it finds on a terminal screen (for which I'm using the ncurses
library).
What I have:
Right now, to do this, I'm calling a function that checks for unsolicited messages in a while loop. The code is roughly akin to:
while (1)
{
// check for messages from PCI and store them in a traffic buffer
checkForMessages(PCIconnection, trafficBuffer);
// output the traffic buffer to the screen
printf("%s", trafficBuffer);
}
What I need:
I need the user to be prompted for input in a way that allows them to end the loop. For example, the user could input end
causing the loop to stop and the program to continue.
The problem:
I'm not aware of a way to achieve this without putting fgets
inside the while loop, causing the program to stop and wait for the user to input something on every loop iteration.
I've looked for a solution, but I haven't been able to find discussion on how to achieve the functionality I need. Opening a new thread or process seems like a step in the right direction?
I'm open to completely restructuring my code if what I'm currently doing is poor practice.
Thank you for any help!
c linux
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Background:
I'm a relatively inexperienced developer trying to write software to interface with a PCI motion controller. I'm using C (compiled with gcc) on Ubuntu Linux 18.04.
The program I'm writing needs to regularly check for unsolicited status messages sent by the motion controller (approx. once per second) and display any messages it finds on a terminal screen (for which I'm using the ncurses
library).
What I have:
Right now, to do this, I'm calling a function that checks for unsolicited messages in a while loop. The code is roughly akin to:
while (1)
{
// check for messages from PCI and store them in a traffic buffer
checkForMessages(PCIconnection, trafficBuffer);
// output the traffic buffer to the screen
printf("%s", trafficBuffer);
}
What I need:
I need the user to be prompted for input in a way that allows them to end the loop. For example, the user could input end
causing the loop to stop and the program to continue.
The problem:
I'm not aware of a way to achieve this without putting fgets
inside the while loop, causing the program to stop and wait for the user to input something on every loop iteration.
I've looked for a solution, but I haven't been able to find discussion on how to achieve the functionality I need. Opening a new thread or process seems like a step in the right direction?
I'm open to completely restructuring my code if what I'm currently doing is poor practice.
Thank you for any help!
c linux
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
Background:
I'm a relatively inexperienced developer trying to write software to interface with a PCI motion controller. I'm using C (compiled with gcc) on Ubuntu Linux 18.04.
The program I'm writing needs to regularly check for unsolicited status messages sent by the motion controller (approx. once per second) and display any messages it finds on a terminal screen (for which I'm using the ncurses
library).
What I have:
Right now, to do this, I'm calling a function that checks for unsolicited messages in a while loop. The code is roughly akin to:
while (1)
{
// check for messages from PCI and store them in a traffic buffer
checkForMessages(PCIconnection, trafficBuffer);
// output the traffic buffer to the screen
printf("%s", trafficBuffer);
}
What I need:
I need the user to be prompted for input in a way that allows them to end the loop. For example, the user could input end
causing the loop to stop and the program to continue.
The problem:
I'm not aware of a way to achieve this without putting fgets
inside the while loop, causing the program to stop and wait for the user to input something on every loop iteration.
I've looked for a solution, but I haven't been able to find discussion on how to achieve the functionality I need. Opening a new thread or process seems like a step in the right direction?
I'm open to completely restructuring my code if what I'm currently doing is poor practice.
Thank you for any help!
c linux
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Background:
I'm a relatively inexperienced developer trying to write software to interface with a PCI motion controller. I'm using C (compiled with gcc) on Ubuntu Linux 18.04.
The program I'm writing needs to regularly check for unsolicited status messages sent by the motion controller (approx. once per second) and display any messages it finds on a terminal screen (for which I'm using the ncurses
library).
What I have:
Right now, to do this, I'm calling a function that checks for unsolicited messages in a while loop. The code is roughly akin to:
while (1)
{
// check for messages from PCI and store them in a traffic buffer
checkForMessages(PCIconnection, trafficBuffer);
// output the traffic buffer to the screen
printf("%s", trafficBuffer);
}
What I need:
I need the user to be prompted for input in a way that allows them to end the loop. For example, the user could input end
causing the loop to stop and the program to continue.
The problem:
I'm not aware of a way to achieve this without putting fgets
inside the while loop, causing the program to stop and wait for the user to input something on every loop iteration.
I've looked for a solution, but I haven't been able to find discussion on how to achieve the functionality I need. Opening a new thread or process seems like a step in the right direction?
I'm open to completely restructuring my code if what I'm currently doing is poor practice.
Thank you for any help!
c linux
c linux
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked 7 hours ago
josephsturmjosephsturm
334
334
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
josephsturm is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Your task requires an event loop based on select
or epoll
. One event it would wait for is user input - when STDIN_FILENO
becomes ready for read. Another is the 1-second periodic timer when you need to poll the controller.
There are quite a few libraries that implement an event loop for you so that you can focus on what events you need to handle and how. libevent
is one of the oldest, feature rich and popular.
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built intoncurses
itself. Calling the functiontimeout
and passing it0
makesgetch
(anncurses
input function) non-blocking, which also solved my problem.
– josephsturm
1 hour ago
add a comment |
Simple answer is multi-threading, where you have thread deployed to wait for user input, while loop continues on. So have this:
char flag = 1;
while (flag) {
// run the loop
// if thing happens deploy the thread which will ask user for input
}
I have not done threading in a while, I think this page would be better than me trying to explain it to you:
https://randu.org/tutorials/threads/
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing listaccu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.
– David C. Rankin
5 hours ago
add a comment |
I believe that the "Unix" way would be not to ask for user input, but to react to a user signal. For example, when the user presses Ctrl-C, the currently running process receives SIGINT.
An example how to properly use SIGINT to interrupt a loop can be found here. Copying it into the answer in case the link gets stale:
#include <stdlib.h>
#include <signal.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
static volatile sig_atomic_t got_signal = 0;
static void my_sig_handler(int signo)
{
got_signal = 1;
}
int main()
{
struct sigaction sa;
memset(&sa, 0, sizeof(struct sigaction));
sa.sa_handler = &my_sig_handler;
if (sigaction(SIGINT, &sa, NULL) == -1) {
perror("sigaction");
return EXIT_FAILURE;
}
for (;;) {
if (got_signal) {
got_signal = 0;
printf("Received interrupt signal!n");
}
printf("Doing useful stuff...n");
sleep(1); /* Sleep is not only useful, it is essential! */
}
return EXIT_SUCCESS;
}
(in your case it would be a good idea to put break;
into the if
block or to use while(!got_signal)
)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
josephsturm is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55660630%2fhow-can-a-c-program-poll-for-user-input-while-simultaneously-performing-other-ac%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your task requires an event loop based on select
or epoll
. One event it would wait for is user input - when STDIN_FILENO
becomes ready for read. Another is the 1-second periodic timer when you need to poll the controller.
There are quite a few libraries that implement an event loop for you so that you can focus on what events you need to handle and how. libevent
is one of the oldest, feature rich and popular.
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built intoncurses
itself. Calling the functiontimeout
and passing it0
makesgetch
(anncurses
input function) non-blocking, which also solved my problem.
– josephsturm
1 hour ago
add a comment |
Your task requires an event loop based on select
or epoll
. One event it would wait for is user input - when STDIN_FILENO
becomes ready for read. Another is the 1-second periodic timer when you need to poll the controller.
There are quite a few libraries that implement an event loop for you so that you can focus on what events you need to handle and how. libevent
is one of the oldest, feature rich and popular.
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built intoncurses
itself. Calling the functiontimeout
and passing it0
makesgetch
(anncurses
input function) non-blocking, which also solved my problem.
– josephsturm
1 hour ago
add a comment |
Your task requires an event loop based on select
or epoll
. One event it would wait for is user input - when STDIN_FILENO
becomes ready for read. Another is the 1-second periodic timer when you need to poll the controller.
There are quite a few libraries that implement an event loop for you so that you can focus on what events you need to handle and how. libevent
is one of the oldest, feature rich and popular.
Your task requires an event loop based on select
or epoll
. One event it would wait for is user input - when STDIN_FILENO
becomes ready for read. Another is the 1-second periodic timer when you need to poll the controller.
There are quite a few libraries that implement an event loop for you so that you can focus on what events you need to handle and how. libevent
is one of the oldest, feature rich and popular.
answered 7 hours ago
Maxim EgorushkinMaxim Egorushkin
90.2k11104192
90.2k11104192
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built intoncurses
itself. Calling the functiontimeout
and passing it0
makesgetch
(anncurses
input function) non-blocking, which also solved my problem.
– josephsturm
1 hour ago
add a comment |
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built intoncurses
itself. Calling the functiontimeout
and passing it0
makesgetch
(anncurses
input function) non-blocking, which also solved my problem.
– josephsturm
1 hour ago
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built into
ncurses
itself. Calling the function timeout
and passing it 0
makes getch
(an ncurses
input function) non-blocking, which also solved my problem.– josephsturm
1 hour ago
Thank you for answering! This is definitely a valid answer to the question I asked, so I'm accepting it. After a lot more reading, I also discovered that there's an answer built into
ncurses
itself. Calling the function timeout
and passing it 0
makes getch
(an ncurses
input function) non-blocking, which also solved my problem.– josephsturm
1 hour ago
add a comment |
Simple answer is multi-threading, where you have thread deployed to wait for user input, while loop continues on. So have this:
char flag = 1;
while (flag) {
// run the loop
// if thing happens deploy the thread which will ask user for input
}
I have not done threading in a while, I think this page would be better than me trying to explain it to you:
https://randu.org/tutorials/threads/
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing listaccu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.
– David C. Rankin
5 hours ago
add a comment |
Simple answer is multi-threading, where you have thread deployed to wait for user input, while loop continues on. So have this:
char flag = 1;
while (flag) {
// run the loop
// if thing happens deploy the thread which will ask user for input
}
I have not done threading in a while, I think this page would be better than me trying to explain it to you:
https://randu.org/tutorials/threads/
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing listaccu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.
– David C. Rankin
5 hours ago
add a comment |
Simple answer is multi-threading, where you have thread deployed to wait for user input, while loop continues on. So have this:
char flag = 1;
while (flag) {
// run the loop
// if thing happens deploy the thread which will ask user for input
}
I have not done threading in a while, I think this page would be better than me trying to explain it to you:
https://randu.org/tutorials/threads/
Simple answer is multi-threading, where you have thread deployed to wait for user input, while loop continues on. So have this:
char flag = 1;
while (flag) {
// run the loop
// if thing happens deploy the thread which will ask user for input
}
I have not done threading in a while, I think this page would be better than me trying to explain it to you:
https://randu.org/tutorials/threads/
answered 7 hours ago


GoxGox
2,42121129
2,42121129
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing listaccu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.
– David C. Rankin
5 hours ago
add a comment |
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing listaccu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.
– David C. Rankin
5 hours ago
1
1
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
But see 5 Big Fat Reasons Why Mutexes Suck Big Time for a few considerations on multi-threading.
– David C. Rankin
6 hours ago
tnx will read this article
– Gox
6 hours ago
tnx will read this article
– Gox
6 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing list
accu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.– David C. Rankin
5 hours ago
Yes, I'd didn't have much of a feel one way or the other about where and what kind of pitfalls were involved in multithreading until that article was posted on the accu-general mailing list
accu-general@accu.org
. It makes some very good points on the inherent inability to validate multithreaded code.– David C. Rankin
5 hours ago
add a comment |
I believe that the "Unix" way would be not to ask for user input, but to react to a user signal. For example, when the user presses Ctrl-C, the currently running process receives SIGINT.
An example how to properly use SIGINT to interrupt a loop can be found here. Copying it into the answer in case the link gets stale:
#include <stdlib.h>
#include <signal.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
static volatile sig_atomic_t got_signal = 0;
static void my_sig_handler(int signo)
{
got_signal = 1;
}
int main()
{
struct sigaction sa;
memset(&sa, 0, sizeof(struct sigaction));
sa.sa_handler = &my_sig_handler;
if (sigaction(SIGINT, &sa, NULL) == -1) {
perror("sigaction");
return EXIT_FAILURE;
}
for (;;) {
if (got_signal) {
got_signal = 0;
printf("Received interrupt signal!n");
}
printf("Doing useful stuff...n");
sleep(1); /* Sleep is not only useful, it is essential! */
}
return EXIT_SUCCESS;
}
(in your case it would be a good idea to put break;
into the if
block or to use while(!got_signal)
)
add a comment |
I believe that the "Unix" way would be not to ask for user input, but to react to a user signal. For example, when the user presses Ctrl-C, the currently running process receives SIGINT.
An example how to properly use SIGINT to interrupt a loop can be found here. Copying it into the answer in case the link gets stale:
#include <stdlib.h>
#include <signal.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
static volatile sig_atomic_t got_signal = 0;
static void my_sig_handler(int signo)
{
got_signal = 1;
}
int main()
{
struct sigaction sa;
memset(&sa, 0, sizeof(struct sigaction));
sa.sa_handler = &my_sig_handler;
if (sigaction(SIGINT, &sa, NULL) == -1) {
perror("sigaction");
return EXIT_FAILURE;
}
for (;;) {
if (got_signal) {
got_signal = 0;
printf("Received interrupt signal!n");
}
printf("Doing useful stuff...n");
sleep(1); /* Sleep is not only useful, it is essential! */
}
return EXIT_SUCCESS;
}
(in your case it would be a good idea to put break;
into the if
block or to use while(!got_signal)
)
add a comment |
I believe that the "Unix" way would be not to ask for user input, but to react to a user signal. For example, when the user presses Ctrl-C, the currently running process receives SIGINT.
An example how to properly use SIGINT to interrupt a loop can be found here. Copying it into the answer in case the link gets stale:
#include <stdlib.h>
#include <signal.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
static volatile sig_atomic_t got_signal = 0;
static void my_sig_handler(int signo)
{
got_signal = 1;
}
int main()
{
struct sigaction sa;
memset(&sa, 0, sizeof(struct sigaction));
sa.sa_handler = &my_sig_handler;
if (sigaction(SIGINT, &sa, NULL) == -1) {
perror("sigaction");
return EXIT_FAILURE;
}
for (;;) {
if (got_signal) {
got_signal = 0;
printf("Received interrupt signal!n");
}
printf("Doing useful stuff...n");
sleep(1); /* Sleep is not only useful, it is essential! */
}
return EXIT_SUCCESS;
}
(in your case it would be a good idea to put break;
into the if
block or to use while(!got_signal)
)
I believe that the "Unix" way would be not to ask for user input, but to react to a user signal. For example, when the user presses Ctrl-C, the currently running process receives SIGINT.
An example how to properly use SIGINT to interrupt a loop can be found here. Copying it into the answer in case the link gets stale:
#include <stdlib.h>
#include <signal.h>
#include <stdio.h>
#include <string.h>
#include <unistd.h>
static volatile sig_atomic_t got_signal = 0;
static void my_sig_handler(int signo)
{
got_signal = 1;
}
int main()
{
struct sigaction sa;
memset(&sa, 0, sizeof(struct sigaction));
sa.sa_handler = &my_sig_handler;
if (sigaction(SIGINT, &sa, NULL) == -1) {
perror("sigaction");
return EXIT_FAILURE;
}
for (;;) {
if (got_signal) {
got_signal = 0;
printf("Received interrupt signal!n");
}
printf("Doing useful stuff...n");
sleep(1); /* Sleep is not only useful, it is essential! */
}
return EXIT_SUCCESS;
}
(in your case it would be a good idea to put break;
into the if
block or to use while(!got_signal)
)
answered 6 hours ago
Kit.Kit.
71069
71069
add a comment |
add a comment |
josephsturm is a new contributor. Be nice, and check out our Code of Conduct.
josephsturm is a new contributor. Be nice, and check out our Code of Conduct.
josephsturm is a new contributor. Be nice, and check out our Code of Conduct.
josephsturm is a new contributor. Be nice, and check out our Code of Conduct.
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f55660630%2fhow-can-a-c-program-poll-for-user-input-while-simultaneously-performing-other-ac%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
J2ic77rvTdw NJ6aUkaKOqOik aJZR,9oysMEuM