In place solution to remove duplicates from a sorted listFinding longest common prefixRemove duplicates from a sorted arrayFind a number which equals to the total number of integers greater than itself in an arrayFirstDuplicate FinderGiven a sorted array nums, remove the duplicates in-placeRemove all occurrences of an element from an array, in placeRemove duplicates from sorted array, in placeHash table solution to twoSumA One-Pass Hash Table Solution to twoSumSplice-merge two sorted lists in Python
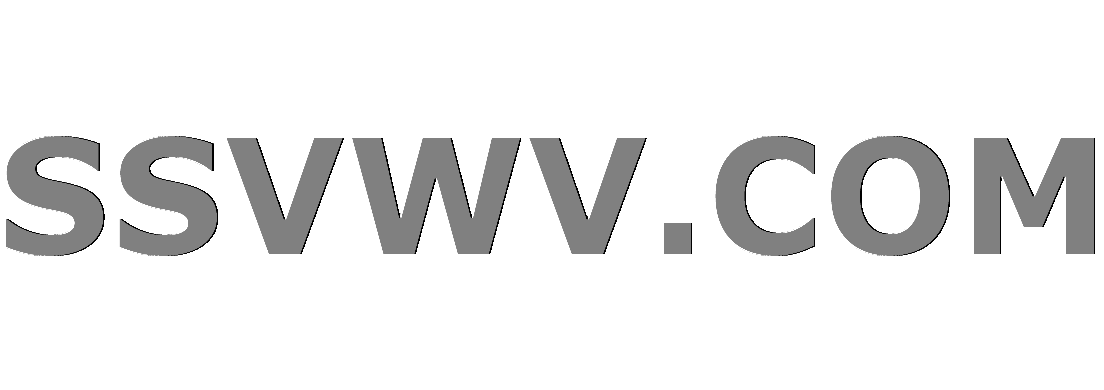
Multi tool use
Was Hulk present at this event?
How did Arya get back her dagger from Sansa?
Why are there synthetic chemicals in our bodies? Where do they come from?
I’ve officially counted to infinity!
Is balancing necessary on a full-wheel change?
Was the ancestor of SCSI, the SASI protocol, nothing more than a draft?
Can a cyclic Amine form an Amide?
Stark VS Thanos
How do you center multiple equations that have multiple steps?
Would "lab meat" be able to feed a much larger global population
If Earth is tilted, why is Polaris always above the same spot?
If an enemy is just below a 10-foot-high ceiling, are they in melee range of a creature on the ground?
Copy line and insert it in a new position with sed or awk
Save terminal output to a txt file
What happens if I start too many background jobs?
Why do freehub and cassette have only one position that matches?
Is it the same airport YUL and YMQ in Canada?
Why was Germany not as successful as other Europeans in establishing overseas colonies?
What are the spoon bit of a spoon and fork bit of a fork called?
Is lying to get "gardening leave" fraud?
Applying a function to a nested list
Pigeonhole Principle Problem
Selecting a secure PIN for building access
Binary Numbers Magic Trick
In place solution to remove duplicates from a sorted list
Finding longest common prefixRemove duplicates from a sorted arrayFind a number which equals to the total number of integers greater than itself in an arrayFirstDuplicate FinderGiven a sorted array nums, remove the duplicates in-placeRemove all occurrences of an element from an array, in placeRemove duplicates from sorted array, in placeHash table solution to twoSumA One-Pass Hash Table Solution to twoSumSplice-merge two sorted lists in Python
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty margin-bottom:0;
$begingroup$
I am working on the problem removeDuplicatesFromSortedList
Given a sorted array nums, remove the duplicates in-place such that each element appear only once and return the new length.
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
Example 1:
Given nums = [1,1,2],
Your function should return length = 2, with the first two elements of nums being 1 and 2 respectively.
It doesn't matter what you leave beyond the returned length.
My solution and TestCase
class Solution:
def removeDuplicates(self, nums: List[int]) -> int:
"""
"""
#Base Case
if len(nums) < 2: return len(nums)
#iteraton Case
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
if nums[j] != nums[i]: #capture the result
i += 1
nums[i] = nums[j] #in place overriden
return i + 1
class MyCase(unittest.TestCase):
def setUp(self):
self.solution = Solution()
def test_raw1(self):
nums = [1, 1, 2]
check = self.solution.removeDuplicates(nums)
answer = 2
self.assertEqual(check, answer)
def test_raw2(self):
nums = [0,0,1,1,1,2,2,3,3,4]
check = self.solution.removeDuplicates(nums)
answer = 5
self.assertEqual(check, answer)
unittest.main()
This runs but I get a report:
Runtime: 72 ms, faster than 49.32% of Python3 online submissions for Remove Duplicates from Sorted Array.
Memory Usage: 14.8 MB, less than 5.43% of Python3 online submissions for Remove Duplicates from Sorted Array.
Less than 5.43%, I employ the in-place strategies but get such a low rank, how could improve it?
python python-3.x programming-challenge memory-optimization
$endgroup$
add a comment |
$begingroup$
I am working on the problem removeDuplicatesFromSortedList
Given a sorted array nums, remove the duplicates in-place such that each element appear only once and return the new length.
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
Example 1:
Given nums = [1,1,2],
Your function should return length = 2, with the first two elements of nums being 1 and 2 respectively.
It doesn't matter what you leave beyond the returned length.
My solution and TestCase
class Solution:
def removeDuplicates(self, nums: List[int]) -> int:
"""
"""
#Base Case
if len(nums) < 2: return len(nums)
#iteraton Case
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
if nums[j] != nums[i]: #capture the result
i += 1
nums[i] = nums[j] #in place overriden
return i + 1
class MyCase(unittest.TestCase):
def setUp(self):
self.solution = Solution()
def test_raw1(self):
nums = [1, 1, 2]
check = self.solution.removeDuplicates(nums)
answer = 2
self.assertEqual(check, answer)
def test_raw2(self):
nums = [0,0,1,1,1,2,2,3,3,4]
check = self.solution.removeDuplicates(nums)
answer = 5
self.assertEqual(check, answer)
unittest.main()
This runs but I get a report:
Runtime: 72 ms, faster than 49.32% of Python3 online submissions for Remove Duplicates from Sorted Array.
Memory Usage: 14.8 MB, less than 5.43% of Python3 online submissions for Remove Duplicates from Sorted Array.
Less than 5.43%, I employ the in-place strategies but get such a low rank, how could improve it?
python python-3.x programming-challenge memory-optimization
$endgroup$
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44
add a comment |
$begingroup$
I am working on the problem removeDuplicatesFromSortedList
Given a sorted array nums, remove the duplicates in-place such that each element appear only once and return the new length.
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
Example 1:
Given nums = [1,1,2],
Your function should return length = 2, with the first two elements of nums being 1 and 2 respectively.
It doesn't matter what you leave beyond the returned length.
My solution and TestCase
class Solution:
def removeDuplicates(self, nums: List[int]) -> int:
"""
"""
#Base Case
if len(nums) < 2: return len(nums)
#iteraton Case
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
if nums[j] != nums[i]: #capture the result
i += 1
nums[i] = nums[j] #in place overriden
return i + 1
class MyCase(unittest.TestCase):
def setUp(self):
self.solution = Solution()
def test_raw1(self):
nums = [1, 1, 2]
check = self.solution.removeDuplicates(nums)
answer = 2
self.assertEqual(check, answer)
def test_raw2(self):
nums = [0,0,1,1,1,2,2,3,3,4]
check = self.solution.removeDuplicates(nums)
answer = 5
self.assertEqual(check, answer)
unittest.main()
This runs but I get a report:
Runtime: 72 ms, faster than 49.32% of Python3 online submissions for Remove Duplicates from Sorted Array.
Memory Usage: 14.8 MB, less than 5.43% of Python3 online submissions for Remove Duplicates from Sorted Array.
Less than 5.43%, I employ the in-place strategies but get such a low rank, how could improve it?
python python-3.x programming-challenge memory-optimization
$endgroup$
I am working on the problem removeDuplicatesFromSortedList
Given a sorted array nums, remove the duplicates in-place such that each element appear only once and return the new length.
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
Example 1:
Given nums = [1,1,2],
Your function should return length = 2, with the first two elements of nums being 1 and 2 respectively.
It doesn't matter what you leave beyond the returned length.
My solution and TestCase
class Solution:
def removeDuplicates(self, nums: List[int]) -> int:
"""
"""
#Base Case
if len(nums) < 2: return len(nums)
#iteraton Case
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
if nums[j] != nums[i]: #capture the result
i += 1
nums[i] = nums[j] #in place overriden
return i + 1
class MyCase(unittest.TestCase):
def setUp(self):
self.solution = Solution()
def test_raw1(self):
nums = [1, 1, 2]
check = self.solution.removeDuplicates(nums)
answer = 2
self.assertEqual(check, answer)
def test_raw2(self):
nums = [0,0,1,1,1,2,2,3,3,4]
check = self.solution.removeDuplicates(nums)
answer = 5
self.assertEqual(check, answer)
unittest.main()
This runs but I get a report:
Runtime: 72 ms, faster than 49.32% of Python3 online submissions for Remove Duplicates from Sorted Array.
Memory Usage: 14.8 MB, less than 5.43% of Python3 online submissions for Remove Duplicates from Sorted Array.
Less than 5.43%, I employ the in-place strategies but get such a low rank, how could improve it?
python python-3.x programming-challenge memory-optimization
python python-3.x programming-challenge memory-optimization
edited Mar 28 at 11:46
Graipher
27.8k54499
27.8k54499
asked Mar 28 at 10:44


AliceAlice
3287
3287
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44
add a comment |
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44
add a comment |
2 Answers
2
active
oldest
votes
$begingroup$
One way that might speed up your solution slightly is to only place the value if necessary. Also note that only one of the two if
conditions can be true, so just use else
. Or even better, since there was a continue
in the other case, just don't indent it.
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = nums[j] #in place overriden
You can also save half of the index lookups by iterating over the values. Of course it will need slightly more memory this way.
def removeDuplicates(nums):
if len(nums) < 2:
return len(nums)
i = 0 #slow-run pointer
for j, value in enumerate(nums):
if value == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = value # in place overriden
return i + 1
This can probably be further sped-up by saving nums[i]
in a variable as well.
What is interesting is to see timing comparisons to using the itertools
recipe unique_justseen
(which I would recommend you use in production if you want to get duplicate free values in vanilla Python):
from itertools import groupby
from operator import itemgetter
def unique_justseen(iterable, key=None):
"List unique elements, preserving order. Remember only the element just seen."
# unique_justseen('AAAABBBCCDAABBB') --> A B C D A B
# unique_justseen('ABBCcAD', str.lower) --> A B C A D
return map(next, map(itemgetter(1), groupby(iterable, key)))
def remove_duplicates(nums):
for i, value in enumerate(unique_justseen(nums)):
nums[i] = value
return i + 1
For nums = list(np.random.randint(100, size=10000))
they take almost the same time:
removeDuplicates
took 0.0023sremove_duplicates
took 0.0026s
$endgroup$
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
add a comment |
$begingroup$
A very minor concern: we have this condition:
if len(nums) < 2: return len(nums)
but none of the included tests exercise it. If we want our testing to be complete, we should have cases with empty and 1-element lists as input.
TBH, I'd reduce that to a simpler condition, and remove the need for one of the tests:
if not nums:
return 0
$endgroup$
add a comment |
Your Answer
StackExchange.ifUsing("editor", function ()
StackExchange.using("externalEditor", function ()
StackExchange.using("snippets", function ()
StackExchange.snippets.init();
);
);
, "code-snippets");
StackExchange.ready(function()
var channelOptions =
tags: "".split(" "),
id: "196"
;
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function()
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled)
StackExchange.using("snippets", function()
createEditor();
);
else
createEditor();
);
function createEditor()
StackExchange.prepareEditor(
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: false,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: null,
bindNavPrevention: true,
postfix: "",
imageUploader:
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
,
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
);
);
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216403%2fin-place-solution-to-remove-duplicates-from-a-sorted-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
$begingroup$
One way that might speed up your solution slightly is to only place the value if necessary. Also note that only one of the two if
conditions can be true, so just use else
. Or even better, since there was a continue
in the other case, just don't indent it.
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = nums[j] #in place overriden
You can also save half of the index lookups by iterating over the values. Of course it will need slightly more memory this way.
def removeDuplicates(nums):
if len(nums) < 2:
return len(nums)
i = 0 #slow-run pointer
for j, value in enumerate(nums):
if value == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = value # in place overriden
return i + 1
This can probably be further sped-up by saving nums[i]
in a variable as well.
What is interesting is to see timing comparisons to using the itertools
recipe unique_justseen
(which I would recommend you use in production if you want to get duplicate free values in vanilla Python):
from itertools import groupby
from operator import itemgetter
def unique_justseen(iterable, key=None):
"List unique elements, preserving order. Remember only the element just seen."
# unique_justseen('AAAABBBCCDAABBB') --> A B C D A B
# unique_justseen('ABBCcAD', str.lower) --> A B C A D
return map(next, map(itemgetter(1), groupby(iterable, key)))
def remove_duplicates(nums):
for i, value in enumerate(unique_justseen(nums)):
nums[i] = value
return i + 1
For nums = list(np.random.randint(100, size=10000))
they take almost the same time:
removeDuplicates
took 0.0023sremove_duplicates
took 0.0026s
$endgroup$
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
add a comment |
$begingroup$
One way that might speed up your solution slightly is to only place the value if necessary. Also note that only one of the two if
conditions can be true, so just use else
. Or even better, since there was a continue
in the other case, just don't indent it.
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = nums[j] #in place overriden
You can also save half of the index lookups by iterating over the values. Of course it will need slightly more memory this way.
def removeDuplicates(nums):
if len(nums) < 2:
return len(nums)
i = 0 #slow-run pointer
for j, value in enumerate(nums):
if value == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = value # in place overriden
return i + 1
This can probably be further sped-up by saving nums[i]
in a variable as well.
What is interesting is to see timing comparisons to using the itertools
recipe unique_justseen
(which I would recommend you use in production if you want to get duplicate free values in vanilla Python):
from itertools import groupby
from operator import itemgetter
def unique_justseen(iterable, key=None):
"List unique elements, preserving order. Remember only the element just seen."
# unique_justseen('AAAABBBCCDAABBB') --> A B C D A B
# unique_justseen('ABBCcAD', str.lower) --> A B C A D
return map(next, map(itemgetter(1), groupby(iterable, key)))
def remove_duplicates(nums):
for i, value in enumerate(unique_justseen(nums)):
nums[i] = value
return i + 1
For nums = list(np.random.randint(100, size=10000))
they take almost the same time:
removeDuplicates
took 0.0023sremove_duplicates
took 0.0026s
$endgroup$
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
add a comment |
$begingroup$
One way that might speed up your solution slightly is to only place the value if necessary. Also note that only one of the two if
conditions can be true, so just use else
. Or even better, since there was a continue
in the other case, just don't indent it.
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = nums[j] #in place overriden
You can also save half of the index lookups by iterating over the values. Of course it will need slightly more memory this way.
def removeDuplicates(nums):
if len(nums) < 2:
return len(nums)
i = 0 #slow-run pointer
for j, value in enumerate(nums):
if value == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = value # in place overriden
return i + 1
This can probably be further sped-up by saving nums[i]
in a variable as well.
What is interesting is to see timing comparisons to using the itertools
recipe unique_justseen
(which I would recommend you use in production if you want to get duplicate free values in vanilla Python):
from itertools import groupby
from operator import itemgetter
def unique_justseen(iterable, key=None):
"List unique elements, preserving order. Remember only the element just seen."
# unique_justseen('AAAABBBCCDAABBB') --> A B C D A B
# unique_justseen('ABBCcAD', str.lower) --> A B C A D
return map(next, map(itemgetter(1), groupby(iterable, key)))
def remove_duplicates(nums):
for i, value in enumerate(unique_justseen(nums)):
nums[i] = value
return i + 1
For nums = list(np.random.randint(100, size=10000))
they take almost the same time:
removeDuplicates
took 0.0023sremove_duplicates
took 0.0026s
$endgroup$
One way that might speed up your solution slightly is to only place the value if necessary. Also note that only one of the two if
conditions can be true, so just use else
. Or even better, since there was a continue
in the other case, just don't indent it.
i = 0 #slow-run pointer
for j in range(1, len(nums)):
if nums[j] == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = nums[j] #in place overriden
You can also save half of the index lookups by iterating over the values. Of course it will need slightly more memory this way.
def removeDuplicates(nums):
if len(nums) < 2:
return len(nums)
i = 0 #slow-run pointer
for j, value in enumerate(nums):
if value == nums[i]:
continue
# capture the result
i += 1
if i != j:
nums[i] = value # in place overriden
return i + 1
This can probably be further sped-up by saving nums[i]
in a variable as well.
What is interesting is to see timing comparisons to using the itertools
recipe unique_justseen
(which I would recommend you use in production if you want to get duplicate free values in vanilla Python):
from itertools import groupby
from operator import itemgetter
def unique_justseen(iterable, key=None):
"List unique elements, preserving order. Remember only the element just seen."
# unique_justseen('AAAABBBCCDAABBB') --> A B C D A B
# unique_justseen('ABBCcAD', str.lower) --> A B C A D
return map(next, map(itemgetter(1), groupby(iterable, key)))
def remove_duplicates(nums):
for i, value in enumerate(unique_justseen(nums)):
nums[i] = value
return i + 1
For nums = list(np.random.randint(100, size=10000))
they take almost the same time:
removeDuplicates
took 0.0023sremove_duplicates
took 0.0026s
edited Mar 28 at 14:38
answered Mar 28 at 11:54
GraipherGraipher
27.8k54499
27.8k54499
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
add a comment |
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity I read it as both improvements would be welcome (and since they accepted the answer, apparently it was not so far off the mark either). Note that answers are free to comment on any and all aspects of the code here, anyways, regardless of what the OP wants.
$endgroup$
– Graipher
Mar 28 at 15:29
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
$begingroup$
@Aethenosity Feel free to post another answer if you see a way to reduce the memory used, though!
$endgroup$
– Graipher
Mar 28 at 15:30
add a comment |
$begingroup$
A very minor concern: we have this condition:
if len(nums) < 2: return len(nums)
but none of the included tests exercise it. If we want our testing to be complete, we should have cases with empty and 1-element lists as input.
TBH, I'd reduce that to a simpler condition, and remove the need for one of the tests:
if not nums:
return 0
$endgroup$
add a comment |
$begingroup$
A very minor concern: we have this condition:
if len(nums) < 2: return len(nums)
but none of the included tests exercise it. If we want our testing to be complete, we should have cases with empty and 1-element lists as input.
TBH, I'd reduce that to a simpler condition, and remove the need for one of the tests:
if not nums:
return 0
$endgroup$
add a comment |
$begingroup$
A very minor concern: we have this condition:
if len(nums) < 2: return len(nums)
but none of the included tests exercise it. If we want our testing to be complete, we should have cases with empty and 1-element lists as input.
TBH, I'd reduce that to a simpler condition, and remove the need for one of the tests:
if not nums:
return 0
$endgroup$
A very minor concern: we have this condition:
if len(nums) < 2: return len(nums)
but none of the included tests exercise it. If we want our testing to be complete, we should have cases with empty and 1-element lists as input.
TBH, I'd reduce that to a simpler condition, and remove the need for one of the tests:
if not nums:
return 0
answered Mar 28 at 16:42


Toby SpeightToby Speight
27.9k742120
27.9k742120
add a comment |
add a comment |
Thanks for contributing an answer to Code Review Stack Exchange!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
Use MathJax to format equations. MathJax reference.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function ()
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fcodereview.stackexchange.com%2fquestions%2f216403%2fin-place-solution-to-remove-duplicates-from-a-sorted-list%23new-answer', 'question_page');
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function ()
StackExchange.helpers.onClickDraftSave('#login-link');
);
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HMP,Nzzu2JB4pSBGthkd8j7m,M74INmoV 8e8rPBvf
$begingroup$
This would be cheating, but did you try if the online judge actually checks if the array is manipulated in-place?
$endgroup$
– Graipher
Mar 28 at 11:44